Building an app is one thing, but guiding users through it? That’s where things can go south.
If users can’t figure out your product fast, they bounce. And let’s face it—no one enjoys digging through help docs or fumbling around an interface. What users need is a clear path that helps them discover value right when they need it.Â
The right product tour creates first impressions, turning confusion into confidence. But not every onboarding tool is compatible with apps built with Angular. Some are a pain to integrate, others feel clunky, and a few don’t offer the customization developers need.
So, how do you find one that works? We walk you through the 8 best Angular onboarding tour libraries, with the pros, quirks, and best use cases for each. Whether you're after a quick plug-and-play tool or something deeply customizable, this guide will help you find the right fit.
Let’s get your users from "What does this button do?" to "This is exactly what I needed."
Library | Pros | Cons | GitHub stars |
Angular Intro.js | ✅ Lightweight (10KB gzipped) with no dependencies ✅ Easy integration with Angular via a dedicated library ✅ Large community (21,000+ GitHub stars) | ❌ Requires JavaScript and CSS skills for customization ❌ Content may break with minor UI changes ❌ Paid license needed for commercial use | 504 |
Angular Shepherd | ✅ Highly responsive design across devices ✅Full keyboard navigation support ✅Uses Floating UI for improved positioning ✅ Framework-specific wrapper for Angular | ❌ Steeper learning curve for complex customizations ❌Lacks built-in user engagement tracking ❌ No official paid support (community-driven) ❌ Potential maintenance challenges for non-technical teams | 218 |
Angular UI Tour | ✅Uses Angular UI Bootstrap's popovers for tour steps ✅ No dependency on jQuery or Twitter Bootstrap ✅ Supports multi-page tours ✅ Allows creation of detached tours | ❌ Requires multiple dependencies (Angular, Angular Sanitize, Angular Hotkeys, etc.) ❌ Limited built-in analytics for tracking tour effectiveness | 92 |
Ngx UI Tour | ✅ Highly responsive design ✅ Easy to set up for Angular 9+ apps ✅ Uses Angular Material MatMenu for displaying tours ✅ Supports multiple positioning options (top, bottom, left, right) ✅ No dependency on jQuery or Twitter Bootstrap | ❌ Requires Angular Material to be installed ❌ Could be overwhelming for simple tour needs ❌ Less suitable for non-Material Design projects | 173 |
Angular Popper | ✅ Lightweight (10KB gzipped) with no dependencies ✅ Handles complex positioning scenarios (scrolling containers, DOM context) ✅ Supports virtual positioning (e.g., following mouse cursor) ✅ Used in popular libraries like Bootstrap and Material UI | ❌ Requires JavaScript skills for implementation and customization ❌ Requires manual integration with Angular (no official wrapper) ❌ Focuses solely on positioning, additional logic needed for tours | 9 |
Angular Joyride | ✅ Supports custom HTML templates for step content ✅ Allows setting custom CSS classes for steps ✅ Includes a built-in progress bar feature ✅ Provides options for tour completion behavior (e.g., redirect URL) ✅ Offers a "waitingTime" option to delay step appearance | ❌ Limited to Angular 9+ versions ❌ No built-in multi-language support; requires manual implementation ❌ No native mobile gesture support (e.g., swipe to navigate) ❌ No built-in A/B testing capabilities for tour variations | 245 |
Material Walk-Through Library | ✅ Ensures consistency across user interfaces ✅ Provides a wide range of pre-built, reusable components ✅ Regular updates and maintenance from the Angular team ✅ Follows Material Design principles for intuitive UX | ❌ Doesn't use semantic HTML, which can affect SEO ❌ Steep learning curve for developers new to Material Design ❌ Lack of real-world examples for CDK components ❌ Can lead to performance issues in large-scale applications | 73 |
Angular Co-Pilot | ✅Provides a unique, funky style for highlighting elements ✅Uses animate.css for improved animation capabilities ✅Allows customization of overlay color through CSS variables ✅Supports template-based content for each step | ❌ May require additional effort to customize beyond basic functionality ❌ Depends on external libraries (animate.css) for full functionality | 82 |
What is an Angular Onboarding Tour?
An Angular onboarding tour is an in-app guide that helps users learn how to use key features in a web application built with Angular, a JavaScript-based front-end framework developed by Google. Â
Angular onboarding tours are implemented using Angular-specific libraries like Angular Shepherd or ngx-ui-tour (more on these later), which allow developers to add interactive guides to the app’s UI.Â
The tour might, for example, highlight the first steps a user needs to take, like:
Setting preferences
Managing tasksÂ
Configuring dashboards—right within the interface
8 Best Angular onboarding tour libraries
Building effective onboarding tours doesn’t have to be time-consuming when you use the right user onboarding tools. For Angular applications, several open-source libraries can help you streamline the process. Here are six libraries worth checking out for building your Angular product tour.
We’ve picked them out based on their ease of use as well as their reliability and how they are being looked after by the team that made them. For instance, we’ve omitted libraries that are not being maintained anymore.Â
1. Angular Intro.jsÂ
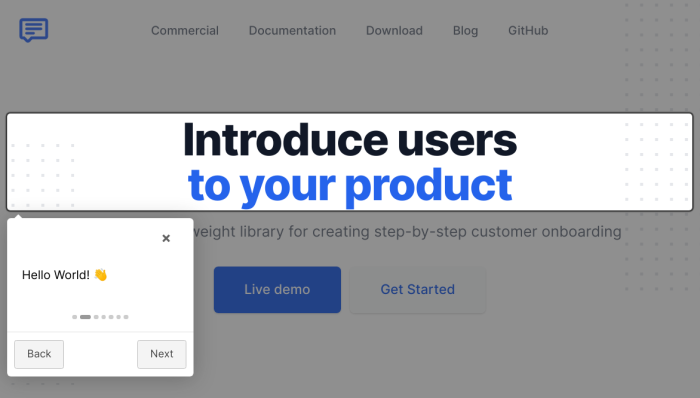
Good old Intro.js. We’ve talked about this tour library for Angular in our previous articles on JavaScript tours, React tours, and Vue.js tours. Of course, Intro.js also works with Angular.Â
Intro.js is a well-known library for creating step-by-step introductions and guided tours on web applications. It is used by major companies like Amazon and SAP, so it is quite reliable and trusted. Unlike various other open-source libraries, Intro.js has a commercial license that starts at a one-time purchase of $9.99.Â
If you decide to get Intro.js, make sure to get the Angular wrapper, which allows Intro.js to work inside an Angular app.Â
Angular Intro.js key user onboarding features
Step-by-step guidance: Intro.js helps you walk users through your app’s features one step at a time, so they can quickly learn and get comfortable without any confusion.
Customizable elements: You can adjust tooltips, modals, and popovers to match your product’s design, providing a seamless user experience.
Responsive to user actions: Intro.js adapts as users interact, allowing you to deliver a relevant experience that meets their needs in real time.
Fast and simple setup: With a lightweight build, Intro.js keeps your app fast and lets developers add tours with ease, so users get a smooth experience.
Angular-friendly: Intro.js integrates seamlessly with Angular, enabling you to create helpful tours without disrupting users’ workflow.
How to set up the Angular Intro.js product walkthrough library
First, install Intro.js with npm or yarn to install the library:
8 Best Angular Onboarding Tour Libraries For Seamless Product Tours
npm install intro.js @types/intro.js --save
Then, import and configure Intro.js in your Angular component to trigger the walkthrough steps.
8 Best Angular Onboarding Tour Libraries For Seamless Product Tours
import * as introJs from 'intro.js';
// ... In your component class
startTour() {
const intro = introJs();
intro.setOptions({
steps: [
{
element: '#your-element-id',
intro: 'This is the first step',
position: 'right'
},
// Add more steps as needed
]
});
intro.start();
}
Finally, call the startTour() function from your HTML template or component logic to initiate the walkthrough.
Check out Intro.js GitHub repository for more information.
2. Angular Shepherd
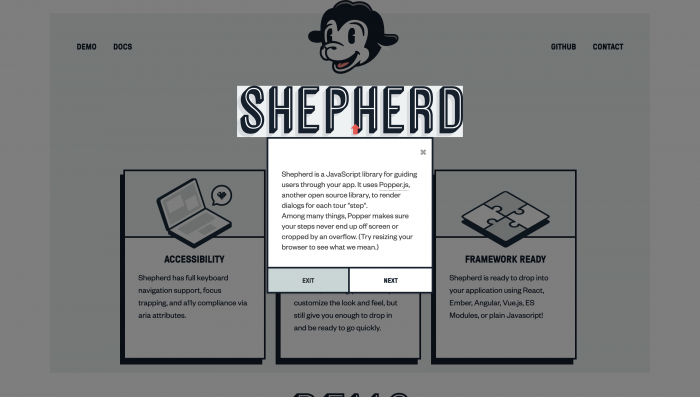
Shepherd is another onboarding library that pretty much works for all JavaScript-based frameworks. Angular as well.
It allows you to define a sequence of steps that guide users through your application's interface, highlighting key elements and providing descriptive tooltips. The library provides a flexible API for customizing the behavior, appearance, and position of each step, as well as the ability to add event handlers for user interactions. Shepherd also provides a wrapper for Angular for quick integration.
Angular Shepherd key user onboarding features
Easily customizable tours: With Shepherd, you can tailor every part of the tour—from tooltips to navigation buttons—so it matches your app’s branding, creating a consistent experience for users.
Contextual user guidance: Shepherd lets you change tour steps based on what users do, enabling you to deliver a more personalized and relevant onboarding experience.
Flexible framework support: While Shepherd integrates seamlessly with Angular, it also works with frameworks like React and Vue, allowing you to adapt tours as your tech stack evolves.
Simple step management: The addSteps() function makes it easy to create and link tour steps to elements, so users can get guided support exactly where they need it.
Focused interaction mode: By dimming the background and highlighting the active element, Shepherd helps users focus on key actions, ensuring they don’t miss important steps.
How to set up the Angular Shepherd product walkthrough library
First, install Angular Shepherd:
8 Best Angular Onboarding Tour Libraries For Seamless Product Tours
npm install angular-shepherd --save
Then, include the Shepherd styles in your project by adding them to your angular.json file:
8 Best Angular Onboarding Tour Libraries For Seamless Product Tours
"styles": ["node_modules/shepherd.js/dist/css/shepherd.css"]
Lastly, import ShepherdService into your component and initialize it with steps to start your tour.
Check out the Shepherd GitHub repository for more information.Â
3. Angular UI TourÂ
Angular UI Tour is a plugin that uses popovers from Angular UI Bootstrap to display a guided tour. This tour library for Angular allows developers to create immersive onboarding experiences by defining custom tour steps and interactive elements that can guide users through key app features or workflows. Designed to blend smoothly with Angular’s framework, it supports routing and other Angular functionalities, making it a great choice for creating user-focused product tours.
Angular UI Tour key user onboarding features
Customizable tooltips and popovers: With Angular UI Tour, you can create engaging tooltips and pop-ups that align perfectly with your app’s look, making tours feel like a natural part of the user experience.
Multi-page tour support: Guide users across different pages, ensuring they get a smooth and complete walkthrough of your app’s features, no matter where they are.
Keyboard-friendly navigation: Users can easily navigate the tour using keyboard shortcuts, like arrow keys, for a more accessible and user-friendly experience.
Backdrop highlighting: This feature highlights key elements by dimming the background, helping users focus on important actions without distractions.
Seamless integration with Angular routers: Angular UI Tour works with Angular’s routing, allowing you to start specific tour steps based on page changes for highly relevant, context-aware guidance.
How to set up the Angular UI Tour product walkthrough library
First, install Angular UI Tour:
8 Best Angular Onboarding Tour Libraries For Seamless Product Tours
npm install angular-ui-tour --save
Then, add the required script tags to your project to enable tour functionality.
Lastly, include the uiTour module in your Angular app:
8 Best Angular Onboarding Tour Libraries For Seamless Product Tours
angular.module('myApp', ['bm.uiTour']);
Check out the Angular UI Tour GitHub repository for more information.
4. Ngx UI Tour
Ngx UI Tour is a versatile Angular library for creating guided tours for onboarding processes, especially suited for apps built with Angular Material. With Angular Material's MatMenu, you can design detailed, interactive tours that guide users through critical areas of your app.Â
Originally developed to replace the now-unmaintained Ngx Tour, Ngx UI Tour stands out with regular updates and support for a range of UI frameworks, ensuring your tours stay current and compatible. Installation is straightforward, but you’ll need Angular Material set up first.
Ngx UI Tour key user onboarding features
Flexibility with multiple UI frameworks: Ngx UI Tour works with Angular Material, Bootstrap, and Ionic, so you can create tours that fit seamlessly within your app’s existing design.
Customizable step templates: Easily customize each tour step with your own content and styles, creating a consistent look that matches your app.
Contextual anchor points: Set anchor points throughout the app to display each tour step in the most relevant spot, giving users clear, context-based guidance.
Keyboard navigation: Users can navigate through the tour with keyboard shortcuts, making it easy for everyone to follow along.
Smooth multi-page guidance: Ngx UI Tour integrates with Angular’s routing, so your tours can flow naturally across different pages, ensuring users never lose their place.
How to set up the Ngx UI Tour product walkthrough library
First, install one of the Ngx UI Tour packages based on your preferred UI framework (e.g., Material or Bootstrap):
8 Best Angular Onboarding Tour Libraries For Seamless Product Tours
npm i --save ngx-ui-tour-md-menu
Then, add the <tour-step-template></tour-step-template> tag to your root component to enable tour steps.
Lastly, define anchor points with the tourAnchor directive throughout your app:
8 Best Angular Onboarding Tour Libraries For Seamless Product Tours
Check out the ngx guided Tour GitHub repository for more information.
5. Angular Popper
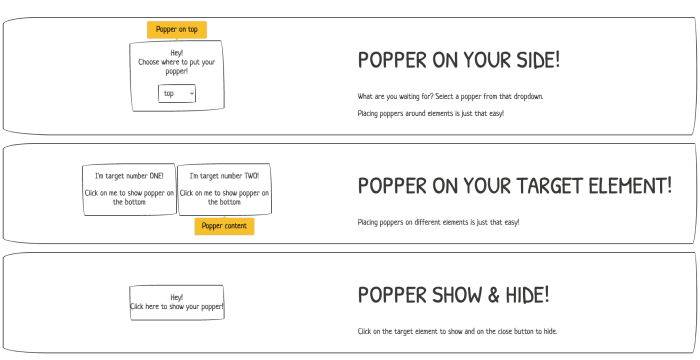
Angular Popper is built on top of Popper.js, which is a lightweight tooltip and popover library itself. This tour library for Angular focuses on creating tooltips. You can position them, arrange them in order, and point them to certain elements. If all you need are great tooltips to give in-app guidance, Angular Popper may be the best fit for you.Â
Angular Popper key user onboarding features
Accurate positioning: Popper keeps tooltips, pop-ups, and dropdowns exactly where they should be, even as users scroll or resize, ensuring everything stays in view.
Easy customization: Adjust placements, alignment, and visibility with Popper’s flexible settings, so your interactive elements fit your app’s design perfectly.
Responsive to user actions: Popper adapts smoothly to different screen sizes and orientations, making sure users can interact with elements effortlessly on any device.
Seamless Angular integration: Popper’s lightweight wrapper works well with Angular, preserving your app’s speed and performance while adding helpful guidance features.
How to set up the Angular Popper product walkthrough library
First, install Angular Popper with NPM:
8 Best Angular Onboarding Tour Libraries For Seamless Product Tours
npm install --save popper.js angular-popper
Then, import NgxPopper into your app module:
8 Best Angular Onboarding Tour Libraries For Seamless Product Tours
import { NgxPopper } from 'angular-popper';
@NgModule({
imports: [NgxPopper],
})
export class AppModule {}
Lastly, use the <angular-popper> component in your templates to define popovers and content placement.
Check out the Angular Popper GitHub repository for more information
6. Angular Joyride
Angular Joyride is a product tour library built entirely in Angular with no external dependencies. You can use custom HTML content, pass parameters to your templates, set up multi-page navigation, and do everything you need to guide users through your site easily.Â
It’s also fairly well maintained. New releases are not as frequent as NgX UI Tour, but the last release was in January 2022. Still fairly recent when compared to some other libraries on this list.Â
Angular Joyride key user onboarding features
Optimized for Angular: Joyride is built fully in Angular without heavy dependencies, so you get smooth performance and reliable compatibility with your Angular app.
Personalized tour steps: Easily customize each step’s position, title, and content, allowing you to create onboarding experiences that feel tailored to your users.
Guided multi-page navigation: Joyride supports tours that move across different routes, helping users navigate seamlessly through various parts of your app.
Consistent design with flexible templates: Use Angular templates for step content, buttons, and counters, so your tours match your app’s design for a cohesive look.
Dynamic control over actions: Trigger specific actions or load elements as users progress through the tour, giving you control over the experience with each step.
How to set up the Angular Joyride product walkthrough library
First, install the library:
8 Best Angular Onboarding Tour Libraries For Seamless Product Tours
npm install ngx-joyride --save
Then, import JoyrideModule into your app module:
8 Best Angular Onboarding Tour Libraries For Seamless Product Tours
import { JoyrideModule } from 'ngx-joyride';
@NgModule({
imports: [JoyrideModule.forRoot(), BrowserModule],
})
export class AppModule {}
Lastly, inject JoyrideService into your component and trigger the tour:
8 Best Angular Onboarding Tour Libraries For Seamless Product Tours
constructor(private joyrideService: JoyrideService) {}
startTour() {
this.joyrideService.startTour({ steps: ['firstStep', 'secondStep'] });
}
Check out the Joyride GitHub repository for more information.
7. Material Walk-Through Library (bdc-walkthrough)
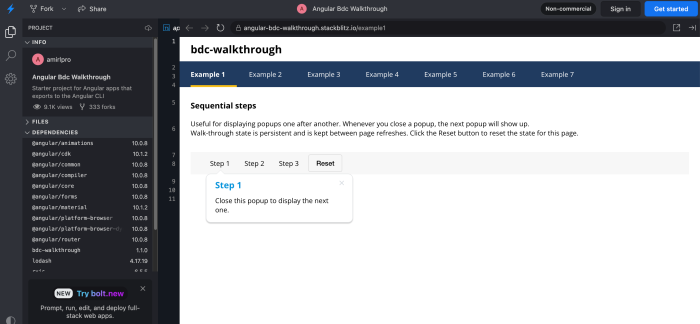
The Material Walk-Through Library (bdc-walkthrough) lets you create guided pop-ups and dialogs within your Angular apps that use Angular Material.Â
A key feature is the BdcWalkService, which tracks completed tasks and stores their state in local storage. This ensures that users only see relevant steps—if a task is marked complete, its pop-up won’t reappear. You can trigger pop-ups based on specific conditions for effective product tours—like form submissions, or set them to show automatically at certain steps in the user journey.
This library also integrates with Angular’s routing system, allowing you to create multi-page tours. You can link steps across different sections of your app, ensuring the tour flows naturally even as users navigate between pages.
🤔Wondering if your product tours are truly engaging users? We analyzed 15 million product tour interactions to find the best practices and actionable insights. Check out our product tour benchmark highlights.
Material Walk-Through Library key user onboarding features
Task-focused guidance: The library uses pop-ups and dialogs triggered by task completion, showing users only the instructions they need at the right time.
Progress tracking: With BdcWalkService, task progress is saved in local storage, so completed steps don’t reappear, keeping the experience smooth and clutter-free.
Continuous multi-page tours: This library works with Angular’s routing, letting users follow guided steps across different sections of your app without interruption.
Customizable triggers for a tailored experience: Control when pop-ups appear based on criteria like task status or user actions, creating a personalized onboarding flow.
Seamless Angular Material design: Built to align with Angular Material, the library blends with your app’s UI for a cohesive, professional look.
How to set up the Material Walk-Through Library
First, install Angular Material:
8 Best Angular Onboarding Tour Libraries For Seamless Product Tours
ng add @angular/material
Then, install the bdc-walkthrough library:
8 Best Angular Onboarding Tour Libraries For Seamless Product Tours
npm install bdc-walkthrough --save
Lastly, import BdcWalkModule into your app.module.ts
Check out the bdc-walkthrough GitHub repository for more information.
8. Angular Co-Pilot (ngx-copilot)
.gif)
The Angular Co-Pilot (ngx-copilot) library helps you build dynamic onboarding tours for Angular applications.Â
The library allows you to define custom templates for each tour step, so the design matches your app’s style. It also includes state management features, letting you track a user’s progress and reinitialize tours at specific steps if needed. The integration with Angular ensures your product walkthroughs don’t disrupt app performance or user experience.
Angular Co-Pilot key user onboarding features
Dynamic step-by-step guidance: build customized tours that walk users through workflows interactively using unique templates.
Smooth navigation control: manage the flow with lifecycle events like next and done, ensuring users transition seamlessly between steps.
Themed overlays and customization: Adjust overlay styles and colors to match your branding
Angular integration: designed to work naturally within Angular projects, maintaining your app's performance and structure
How to set up the Angular Co-Pilot product walkthrough library
First, install the required packages:
8 Best Angular Onboarding Tour Libraries For Seamless Product Tours
npm install ngx-copilot@latest --save
npm install animate.css --save
Then, add the styles to angular.json:
8 Best Angular Onboarding Tour Libraries For Seamless Product Tours
"styles": [
"./node_modules/animate.css/animate.min.css",
"./node_modules/ngx-copilot/src/lib/ngx-copilot.css"
]
Lastly, import the NgxCopilotModule in app.module.ts
8 Best Angular Onboarding Tour Libraries For Seamless Product Tours
import { NgxCopilotModule } from 'ngx-copilot';
@NgModule({
imports: [NgxCopilotModule],
})
export class AppModule {}
Use the <ngx-wrapper-copilot> component in your templates to define the steps and control tour behavior.
Check out the Angular Co-Pilot GitHub repository for more information.
Don’t know how to code? Here’s a no-code alternative to Angular product tour libraries?
Every hour spent building your own onboarding tours is an hour taken away from scaling your product. While Angular onboarding libraries offer flexibility and control, they also come with a steep learning curve, ongoing maintenance needs, and a time investment.
Instead, consider using a product adoption platform, like Chameleon! With tools like Tours, Tooltips, Microsurveys, and Launchers, you can deliver seamless, on-brand onboarding experiences without relying on developers. Plus, Chameleon’s built-in analytics help you continuously refine and improve user engagement.
Just ask Chili Piper. They used Chameleon to optimize their upsell process and generated $150K+ in ARR in just a few weeks!
Want to turn your in-app experiences into opportunities for growth?
👉 Check out this interactive demo on building an onboarding Launcher in Chameleon.